Sub sub1()
Stri = "abcxyghixy"
substr = "xy"
MsgBox Replace(Stri, substr, "")
End Sub
Friday, October 8, 2010
How do you test a Notepad?
Humans seem to have a natural tendency to categorize. Software Test Engineers are no exception, especially when it comes into breaking down our work items. Different “types” of Testing include the following:
* Functional Testing
* Specification Testing
* Security Testing
* Regression Testing
* Automation Testing
* Beta Testing
* Functional Testing
* Specification Testing
* Security Testing
* Regression Testing
* Automation Testing
* Beta Testing
When is a product ready to ship?
When test is confident that all issues have been identified, documented, and resolved. [A poor answer would be “when all bugs have been fixed”. Upper management is responsible for deciding when a product is ready to ship. It’s tests responsibility to make sure upper management has all the information they need to make a calculated decision.]
What are the different test categories that test cases are commonly grouped by? or Web Testing Methods ?
For a web application
Functionality, Usability, Accessibility, Performance, Stress, Load, Scalability, Security, Compatibility, Localization, Globalization, UI, Documentation,
Functionality, Usability, Accessibility, Performance, Stress, Load, Scalability, Security, Compatibility, Localization, Globalization, UI, Documentation,
What goes into the body of a good bug report?
A good bug report contains a descriptive summary of the bug, the steps to reproduce the bug,screenshots of the bug, the expected behavior of the system, and the actual behavior of the system.
What is the difference between priority and severity?
Priority refers to how important a bug is to be fixed, and severity refers to how detrimental or damaging the bug is to the system or user experience.
Thursday, October 7, 2010
What is Value type and Reference type ? Whats the difference between Struct and class ?
A struct is a value type. To help understand the struct, it's helpful to make a comparison with classes.While a struct is a value type, a class is a reference type. Value types hold their value in memory where they are declared, but reference types hold a reference to an object in memory. If you copy a struct, C# creates a new copy of the object and assigns the copy of the object to a separate struct instance. However, if you copy a class, C# creates a new copy of the reference to the object and assigns the copy of the reference to the separate class instance. Structs can't have destructors, but classes can have destructors. Another difference between a struct and class is that a struct can't have implementation inheritance, but a class can. Although a struct can't have implementation inheritance, it can have interface inheritance.
what is the testing approach for web based application?
Why testing Web Applications is different?
Testing web applications is different because of many factors scenarios affecting the performance and user experience.
Web applications can typically cater to a large and a diverse audience. Web Applications can also be exposed to wide range of security threats. Web applications may open up illegal points of entry to the databases and other systems holding sensitive information.
Test Approach is a part of test plan.Basically this is what you want to test about the application.
1.what are the types of testings that we are going to perform on that application.let us say
1) Functionality Testing
2) Usability testing
3) Interface testing
4) Compatibility testing
5) Performance testing
6) Security testing
http://www.softwaretestinghelp.com/web-application-testing/
2.
Testing web applications is different because of many factors scenarios affecting the performance and user experience.
Web applications can typically cater to a large and a diverse audience. Web Applications can also be exposed to wide range of security threats. Web applications may open up illegal points of entry to the databases and other systems holding sensitive information.
Test Approach is a part of test plan.Basically this is what you want to test about the application.
1.what are the types of testings that we are going to perform on that application.let us say
1) Functionality Testing
2) Usability testing
3) Interface testing
4) Compatibility testing
5) Performance testing
6) Security testing
http://www.softwaretestinghelp.com/web-application-testing/
2.
Gloabalization and Localization Testing
Localization testing checks how well the build has been translated into a particular target language. This test is based on the results of globalized testing where the functional support for that particular locale has already been verified. If the product is not globalized enough to support a given language, you probably will not try to localize it into that language in the first place!
You should be aware that pseudo-localization, which was discussed earlier, does not completely eliminate the need for functionality testing of a localized application. When you test for localizability before you localize, the chances of having serious functional problems due to localization are slim. However, you still have to check that the application you're shipping to a particular market really works. Now you can do it in less time and with fewer resources. The following section shows you some of the general areas on which to focus when performing a localization test. (For information on more specific areas, see "Localization Testing of the UI" later in this article.)
General Areas of Focus in Localization TestingLocalization testing should focus on several general areas. The first involves things that are often altered during localization, such as the UI and content files. The second consists of culture-specific, language-specific, and country-specific areas. Examples include configurable components-such as region defaults and the default language-as well as language-specific and region-specific functionality-such as default spelling checkers, speech engines, and so on. You should also test the availability of drivers for local hardware and look for the encryption algorithms incorporated into the application. The rules and regulations for distribution of cryptographic software differ from country to country.
Pay specific attention to the customization that could not be automated through the globalization services infrastructure (Win32 NLS APIs and the .NET Framework). For example, check that formatting of mailing addresses is locale-specific and that parts of the user's name are ordered correctly. (The order in which surname and first name appear varies according to country. For instance, some Muslim countries and certain regions in India use a different name order than that used in the English language.) Functionality of this kind is often implemented by an application-testing must verify its correctness.
Other areas of localization testing should include basic functionality tests; setup, upgrade, and uninstall tests that are run in the localized environment; and, finally, application and hardware compatibility tests that are planned according to the product's target market.
Platform in Localization TestingAny language version of Windows XP or Windows 2000 can be selected as a platform for the test if the product is properly globalized. Of course, in the case of localization testing, the localized version of the operating system can be a wise choice, since that's the most likely environment for your application in the real world. However, a globalized and localizable application, even after it undergoes localization, must be able to run on any language version of the operating system and with MUI installed.
You should run the application with MUI installed when your application implements an MUI behavior, through pluggable UI, satellite dynamic-link libraries (DLLs), or some other technique that adjusts the UI language to the user's preferences. MUI allows the user to switch the UI language of the operating system and thus you must make sure your application matches the operating-system settings. You should verify the behavior of the application when the user's default language of the UI differs from the other locale settings. By doing so, you'll immediately see any problems in the way resources are loaded and processed.
Localization Testing of the UIAlso keep an eye on the behavior of applications that run processes in a system-such as operating-system services-rather than in a user's context. When a system process queries its user default UI language settings, it might get a result different from what a user's process running at the same time will get. This can cause localization problems, inconsistency in the UI that the user sees (if parts of it are generated by the system services), or even problems in functionality. In order to avoid those problems, always check an application's behavior with different default user and system UI languages. The settings for UI languages should also be different from those used in the development environment.
For example, assume you have a machine with MUI installed and a user whose default UI language is different from that of the system. Suppose a fax service waiting for incoming calls is running continuously and that, when a fax arrives, the service displays a notification message to the currently logged user (if there is one). You must ensure that the message be in the user's language, which might not necessarily be the same as the one returned to the fax service when it queries its default UI language.
In particular, localization testing of the UI and linguistics should cover items such as:
Validation of all application resources.
Verification of linguistic accuracy and resource attributes.
Checking for typographical errors.
Checking that printed documentation, online Help, messages, interface resources, and command-key sequences are consistent with each other. If you have shipped localized versions of your product before, make sure that the translation is consistent with the earlier released versions.
Confirmation of adherence to system, input, and display environment standards.
Checking usability of the UI.
Assessment of cultural appropriateness.
Checking for politically sensitive content.
Making sure the market-specific information about your company, such as contact information or local product-support phone numbers, is updated.
It's also a good idea to check that everything you are going to distribute in a local market complies with the local laws and regulations. (See Designing a World-Ready Program.) This includes not only the license agreement but also online Help and other user documentation.
You've now seen the importance of globalized testing, localizability testing, and localization testing in terms of ensuring both that a product is world-ready and that it is suitable for worldwide distribution. One way in which the testing process has changed to reflect new goals involves the type of tools currently being used.
http://msdn.microsoft.com/en-us/goglobal/bb688151.aspx
You should be aware that pseudo-localization, which was discussed earlier, does not completely eliminate the need for functionality testing of a localized application. When you test for localizability before you localize, the chances of having serious functional problems due to localization are slim. However, you still have to check that the application you're shipping to a particular market really works. Now you can do it in less time and with fewer resources. The following section shows you some of the general areas on which to focus when performing a localization test. (For information on more specific areas, see "Localization Testing of the UI" later in this article.)
General Areas of Focus in Localization TestingLocalization testing should focus on several general areas. The first involves things that are often altered during localization, such as the UI and content files. The second consists of culture-specific, language-specific, and country-specific areas. Examples include configurable components-such as region defaults and the default language-as well as language-specific and region-specific functionality-such as default spelling checkers, speech engines, and so on. You should also test the availability of drivers for local hardware and look for the encryption algorithms incorporated into the application. The rules and regulations for distribution of cryptographic software differ from country to country.
Pay specific attention to the customization that could not be automated through the globalization services infrastructure (Win32 NLS APIs and the .NET Framework). For example, check that formatting of mailing addresses is locale-specific and that parts of the user's name are ordered correctly. (The order in which surname and first name appear varies according to country. For instance, some Muslim countries and certain regions in India use a different name order than that used in the English language.) Functionality of this kind is often implemented by an application-testing must verify its correctness.
Other areas of localization testing should include basic functionality tests; setup, upgrade, and uninstall tests that are run in the localized environment; and, finally, application and hardware compatibility tests that are planned according to the product's target market.
Platform in Localization TestingAny language version of Windows XP or Windows 2000 can be selected as a platform for the test if the product is properly globalized. Of course, in the case of localization testing, the localized version of the operating system can be a wise choice, since that's the most likely environment for your application in the real world. However, a globalized and localizable application, even after it undergoes localization, must be able to run on any language version of the operating system and with MUI installed.
You should run the application with MUI installed when your application implements an MUI behavior, through pluggable UI, satellite dynamic-link libraries (DLLs), or some other technique that adjusts the UI language to the user's preferences. MUI allows the user to switch the UI language of the operating system and thus you must make sure your application matches the operating-system settings. You should verify the behavior of the application when the user's default language of the UI differs from the other locale settings. By doing so, you'll immediately see any problems in the way resources are loaded and processed.
Localization Testing of the UIAlso keep an eye on the behavior of applications that run processes in a system-such as operating-system services-rather than in a user's context. When a system process queries its user default UI language settings, it might get a result different from what a user's process running at the same time will get. This can cause localization problems, inconsistency in the UI that the user sees (if parts of it are generated by the system services), or even problems in functionality. In order to avoid those problems, always check an application's behavior with different default user and system UI languages. The settings for UI languages should also be different from those used in the development environment.
For example, assume you have a machine with MUI installed and a user whose default UI language is different from that of the system. Suppose a fax service waiting for incoming calls is running continuously and that, when a fax arrives, the service displays a notification message to the currently logged user (if there is one). You must ensure that the message be in the user's language, which might not necessarily be the same as the one returned to the fax service when it queries its default UI language.
In particular, localization testing of the UI and linguistics should cover items such as:
Validation of all application resources.
Verification of linguistic accuracy and resource attributes.
Checking for typographical errors.
Checking that printed documentation, online Help, messages, interface resources, and command-key sequences are consistent with each other. If you have shipped localized versions of your product before, make sure that the translation is consistent with the earlier released versions.
Confirmation of adherence to system, input, and display environment standards.
Checking usability of the UI.
Assessment of cultural appropriateness.
Checking for politically sensitive content.
Making sure the market-specific information about your company, such as contact information or local product-support phone numbers, is updated.
It's also a good idea to check that everything you are going to distribute in a local market complies with the local laws and regulations. (See Designing a World-Ready Program.) This includes not only the license agreement but also online Help and other user documentation.
You've now seen the importance of globalized testing, localizability testing, and localization testing in terms of ensuring both that a product is world-ready and that it is suitable for worldwide distribution. One way in which the testing process has changed to reflect new goals involves the type of tools currently being used.
http://msdn.microsoft.com/en-us/goglobal/bb688151.aspx
Tuesday, October 5, 2010
How to test an application which has DataBase Upgrade
1. Identify and execute the testcases which can exercise all the database operations from application.
2. Execute automation scripts
3. Execute performance tests
4. Identify and execute testcases which update the existing records to test the backward compatibilty.
5. Ensure data integrity is maintained after upgrade. Contstraints for example should still be handled as expected.
2. Execute automation scripts
3. Execute performance tests
4. Identify and execute testcases which update the existing records to test the backward compatibilty.
5. Ensure data integrity is maintained after upgrade. Contstraints for example should still be handled as expected.
Monday, October 4, 2010
Contents of the Test Plan :
1.Objective : explains the purposoe of the testing
2.Scope : Explains the limittions of the testing
3.Activites: The actions need to be performed while testing.
4.Dependencies : Explains the prerequisites of the testing
5.Deliverables : The outcomes of the testing
6.H/W&S/w: Contains a list of H/W and S/W reqs
7.Resources: persons reqd,how long they reqd,what skills they shud have
8.Risk FActor : %of risk
9.Test Strategy : The strategy to be followed while tesing
10.Tracebility Matrix : it will have the reqs one side and the test cases other side
2.Scope : Explains the limittions of the testing
3.Activites: The actions need to be performed while testing.
4.Dependencies : Explains the prerequisites of the testing
5.Deliverables : The outcomes of the testing
6.H/W&S/w: Contains a list of H/W and S/W reqs
7.Resources: persons reqd,how long they reqd,what skills they shud have
8.Risk FActor : %of risk
9.Test Strategy : The strategy to be followed while tesing
10.Tracebility Matrix : it will have the reqs one side and the test cases other side
Difference between Smoke Testing and SanityTesting
Smoke testing originated in the hardware testing practice of turning on a new piece of hardware for the first time and considering it a success if it does not catch fire and smoke. In software industry, smoke testing is a shallow and wide approach whereby all areas of the application without getting into too deep, is tested.
A sanity test is a narrow regression test that focuses on one or a few areas of functionality. Sanity testing is usually narrow and deep.
Smoke Testing:
Smoke testing is done at the start of the application is deployed. Smoke test is the entry point for the entire test execution. When the application is passed under the smoke test then only further system testing or regression testing can be carried out.
In general smoke testing is done when the higher version of the build is deployed and is done at each and every time the build is deployed. In smoke testing the main functionalites are tested and the stability of the system is validated.
Sanity Testing:
Sanity testing is also similar to Smoke testing, but has some minor differences. Sanity testing is done when the application is deployed into testing for the very first time and in smoke testing only positive scenarios are validated but in sanity testing both the positive and negative scenarios are validated.
For example, if the new software is crashing systems every 5 minutes, bogging down systems to a crawl, or destroying databases, the software may not be in a 'sane' enough condition to warrant further testing in its current state.
Sanity testing:We will check the complete functionality of the application
Smoke Testing: We will check the basic functionality of the application
A sanity test is a narrow regression test that focuses on one or a few areas of functionality. Sanity testing is usually narrow and deep.
Smoke Testing:
Smoke testing is done at the start of the application is deployed. Smoke test is the entry point for the entire test execution. When the application is passed under the smoke test then only further system testing or regression testing can be carried out.
In general smoke testing is done when the higher version of the build is deployed and is done at each and every time the build is deployed. In smoke testing the main functionalites are tested and the stability of the system is validated.
Sanity Testing:
Sanity testing is also similar to Smoke testing, but has some minor differences. Sanity testing is done when the application is deployed into testing for the very first time and in smoke testing only positive scenarios are validated but in sanity testing both the positive and negative scenarios are validated.
For example, if the new software is crashing systems every 5 minutes, bogging down systems to a crawl, or destroying databases, the software may not be in a 'sane' enough condition to warrant further testing in its current state.
Sanity testing:We will check the complete functionality of the application
Smoke Testing: We will check the basic functionality of the application
Tuesday, August 10, 2010
SQL:List the employees whose salary is greater than their department avg salary
select * from emp e where sal > (select avg(sal) from emp where deptno=e.deptno);
SQL:List the employees who are managers
select * from emp where empno in (select distinct(mgr) from emp);
Thursday, July 22, 2010
What is entry and exit criteria of a test plan?
Entry criteria denotes the conditions or process that must be present before a process can begin.
* All developed code must be unit tested. Unit and Integration Testing must be completed and signed off by development team.
* System Test plans must be signed off by Business Analyst and Test Controller.
* All human resources must be assigned and in place.
* All test hardware and environments must be in place, and free for System test use.
* The Acceptance Tests must be completed, with a pass rate of not less than 80%.
* Build should be ready, testcases should be ready.
Exit criteria as the name implies denotes the conditions or process that must be present before a cycle completes.
*Bug Reports,Test results or Test Summary Report, Test Logs are some of the Exit criteria out of the testing process.
*All High Priority errors from System Test must be fixed and tested.
*If any medium or low-priority errors are outstanding - the implementation risk must be signed off as acceptable by Business Analyst and Business Expert.
*Project Integration Test must be signed off by Test Controller and Business Analyst.
*Business Acceptance Test must be signed off by Business Expert.
* All developed code must be unit tested. Unit and Integration Testing must be completed and signed off by development team.
* System Test plans must be signed off by Business Analyst and Test Controller.
* All human resources must be assigned and in place.
* All test hardware and environments must be in place, and free for System test use.
* The Acceptance Tests must be completed, with a pass rate of not less than 80%.
* Build should be ready, testcases should be ready.
Exit criteria as the name implies denotes the conditions or process that must be present before a cycle completes.
*Bug Reports,Test results or Test Summary Report, Test Logs are some of the Exit criteria out of the testing process.
*All High Priority errors from System Test must be fixed and tested.
*If any medium or low-priority errors are outstanding - the implementation risk must be signed off as acceptable by Business Analyst and Business Expert.
*Project Integration Test must be signed off by Test Controller and Business Analyst.
*Business Acceptance Test must be signed off by Business Expert.
what is the difference between action and function?
1.Actions are QTP specific.whereas functions are vb specific.
2.Actions - can only accept primative data types as Parameters (strings, integers, etc)
Functions - can accept arrays, dictionary objects and test objects (i.e. Pages, Frames, WebRadioGroups, etc.)
3.Actions - Return values are difficult to understand and read in the syntax.
Functions - Return values work like they do in other languages.
4.Actions - actions are associated with object Repositry (Per action or shared dependnds).
Functions : functions don't have object repositry associated with them.
2.Actions - can only accept primative data types as Parameters (strings, integers, etc)
Functions - can accept arrays, dictionary objects and test objects (i.e. Pages, Frames, WebRadioGroups, etc.)
3.Actions - Return values are difficult to understand and read in the syntax.
Functions - Return values work like they do in other languages.
4.Actions - actions are associated with object Repositry (Per action or shared dependnds).
Functions : functions don't have object repositry associated with them.
Wednesday, July 21, 2010
Find a Cell with Specified Text and Click It
Description
Returns the number of the first row found that contains a cell with the specified text.
Syntax
object.GetRowWithCellText (Text, [Column], [StartFromRow])
Argument Description
object A test object of type WebTable.
Text Required. A String value. The text string for which to search.
Column Optional. A Variant value. The column number where the cell is located. The value can either be the column name or index. Index values begin with 1.
StartFromRow Optional. A Long value. The number of the row in which to start the search. The first row in the table is numbered 1.
Return Value
A Long value.
Sub GetRowWithCellText_Example()
'The following example uses the GetRowWithCellText method to
'find the row in the "OutboundFlights" table that contains the
'text "Price" and then find the cell in that row that contains
'a link and click it.
row = Browser("Mercury Tours").Page("Search Results").WebTable("OutboundFlights").GetRowWithCellText("Price", 2, 2)
Set Link = Browser("Mercury Tours").Page("Search Results").WebTable("OutboundFlights").ChildItem(row, 2, "Link", 0)
Link.Click
End Sub
Returns the number of the first row found that contains a cell with the specified text.
Syntax
object.GetRowWithCellText (Text, [Column], [StartFromRow])
Argument Description
object A test object of type WebTable.
Text Required. A String value. The text string for which to search.
Column Optional. A Variant value. The column number where the cell is located. The value can either be the column name or index. Index values begin with 1.
StartFromRow Optional. A Long value. The number of the row in which to start the search. The first row in the table is numbered 1.
Return Value
A Long value.
Sub GetRowWithCellText_Example()
'The following example uses the GetRowWithCellText method to
'find the row in the "OutboundFlights" table that contains the
'text "Price" and then find the cell in that row that contains
'a link and click it.
row = Browser("Mercury Tours").Page("Search Results").WebTable("OutboundFlights").GetRowWithCellText("Price", 2, 2)
Set Link = Browser("Mercury Tours").Page("Search Results").WebTable("OutboundFlights").ChildItem(row, 2, "Link", 0)
Link.Click
End Sub
Tuesday, July 20, 2010
Difference between atendoffile and atendofstream
The difference between AtEndOfline and AtEndOfstream is :
AtEndOfline reads till it encounters a new line.
AtEndOfstream reads till end of the file.
Dim fso, MyFile
Set fso = CreateObject("Scripting.FileSystemObject")
Set MyFile= fso.CreateTextFile("C:\TEMP\testfile.txt", True)
MyFile.WriteLine("This is a test1.")
MyFile.WriteLine("This is a test2."+vbnewline)
MyFile.WriteLine("This is a test3.")
MyFile.Close
AtEndOfline
Const ForReading = 1
Set fso = CreateObject("Scripting.FileSystemObject")
Set theFile = fso.OpenTextFile("C:\TEMP\testfile.txt", ForReading, False)
Do While theFile.AtEndOfline <> True
MSGBOX theFile.Readline
Loop
theFile.Close
returns the output as
This is a test1.
This is a test2.
AtEndOfStream
Const ForReading = 1
Set fso = CreateObject("Scripting.FileSystemObject")
Set theFile = fso.OpenTextFile("C:\TEMP\testfile.txt", ForReading, False)
Do While theFile.AtEndOfstream <> True
MSGBOX theFile.Readline
Loop
theFile.Close
returns the output as
This is a test1.
This is a test2.
This is a test3.
AtEndOfline reads till it encounters a new line.
AtEndOfstream reads till end of the file.
Dim fso, MyFile
Set fso = CreateObject("Scripting.FileSystemObject")
Set MyFile= fso.CreateTextFile("C:\TEMP\testfile.txt", True)
MyFile.WriteLine("This is a test1.")
MyFile.WriteLine("This is a test2."+vbnewline)
MyFile.WriteLine("This is a test3.")
MyFile.Close
AtEndOfline
Const ForReading = 1
Set fso = CreateObject("Scripting.FileSystemObject")
Set theFile = fso.OpenTextFile("C:\TEMP\testfile.txt", ForReading, False)
Do While theFile.AtEndOfline <> True
MSGBOX theFile.Readline
Loop
theFile.Close
returns the output as
This is a test1.
This is a test2.
AtEndOfStream
Const ForReading = 1
Set fso = CreateObject("Scripting.FileSystemObject")
Set theFile = fso.OpenTextFile("C:\TEMP\testfile.txt", ForReading, False)
Do While theFile.AtEndOfstream <> True
MSGBOX theFile.Readline
Loop
theFile.Close
returns the output as
This is a test1.
This is a test2.
This is a test3.
Looping Statements
Looping statements are used to run the same block of code a specified number of times.
In VBScript we have four looping statements:
For...Next statement - runs code a specified number of times
For Each...Next statement - runs code for each item in a collection or each element of an array
Do...Loop statement - loops while or until a condition is true
While...Wend statement - Do not use it - use the Do...Loop statement instead
In VBScript we have four looping statements:
For...Next statement - runs code a specified number of times
For Each...Next statement - runs code for each item in a collection or each element of an array
Do...Loop statement - loops while or until a condition is true
While...Wend statement - Do not use it - use the Do...Loop statement instead
Important Datetime functions
msgbox day(now())
msgbox weekdayname(weekday(now()))
or
msgbox weekdayname (weekday(day(now())))
msgbox monthname(month(now()))
msgbox year(now())
msgbox hour(now)
msgbox minute(now)
msgbox Second(now)
msgbox weekdayname(weekday(now()))
or
msgbox weekdayname (weekday(day(now())))
msgbox monthname(month(now()))
msgbox year(now())
msgbox hour(now)
msgbox minute(now)
msgbox Second(now)
reading environment variable values from the excel vba
Environ Function
Returns the String associated with an operating system environment variable. Not available on the Macintosh
Syntax
Environ({envstring | number})
The Environ function syntax has these named arguments:
Part Description
envstring Optional. String expression containing the name of an environment variable.
number Optional. Numeric expression corresponding to the numeric order of the environment string in the environment-string table. The number argument can be any numeric expression, but is rounded to a whole number before it is evaluated.
Remarks
If envstring can't be found in the environment-string table, a zero-length string ("") is returned. Otherwise, Environ returns the text assigned to the specified envstring; that is, the text following the equal sign (=) in the environment-string table for that environment variable.
If you specify number, the string occupying that numeric position in the environment-string table is returned. In this case, Environ returns all of the text, including envstring. If there is no environment string in the specified position, Environ returns a zero-length string.
Sub List_Environment_Variables()
Dim EnvString As String
Indx = 1
Do
EnvString = Environ(Indx)
Cells(Indx, 1) = EnvString
Indx = Indx + 1
Loop Until EnvString = ""
End Sub
ALLUSERSPROFILE=C:\Documents and Settings\All Users
APPDATA=C:\Documents and Settings\nbdyvek\Application Data
CommonProgramFiles=C:\Program Files\Common Files
COMPUTERNAME=B0024811EE6F0
ComSpec=C:\WINNT\system32\cmd.exe
DEFLOGDIR=C:\Documents and Settings\All Users\Application Data\McAfee\DesktopProtection
FP_NO_HOST_CHECK=NO
HOMEDRIVE=C:
HOMEPATH=\Documents and Settings\nbdyvek
LOGONSERVER=\\ASIGGNDCS00
LSERVRC=C:\Program Files\Common Files\Mercury Interactive\License Manager\lservrc
LSHOST=172.23.3.194
NUMBER_OF_PROCESSORS=4
OS=Windows_NT
Path=C:\Program Files\Microsoft Office\Office12\;c:\Program Files\oracle\product\10.2.0\client_1\bin;C:\WINNT\system32;C:\WINNT;C:\WINNT\System32\Wbem;"C:\Program Files\PKWARE\pkzipc"
PATHEXT=.COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH
PROCESSOR_ARCHITECTURE=x86
PROCESSOR_IDENTIFIER=x86 Family 6 Model 23 Stepping 10, GenuineIntel
PROCESSOR_LEVEL=6
PROCESSOR_REVISION=170a
ProgramFiles=C:\Program Files
SESSIONNAME=Console
SystemDrive=C:
SystemRoot=C:\WINNT
TEMP=C:\DOCUME~1\nbdyvek\LOCALS~1\Temp
TMP=C:\DOCUME~1\nbdyvek\LOCALS~1\Temp
USERDNSDOMAIN=ASIA.BANKOFAMERICA.COM
USERDOMAIN=ASIA
USERNAME=nbdyvek
USERPROFILE=C:\Documents and Settings\nbdyvek
VSEDEFLOGDIR=C:\Documents and Settings\All Users\Application Data\McAfee\DesktopProtection
WecVersionForRosebud.E88=3
windir=C:\WINNT
Returns the String associated with an operating system environment variable. Not available on the Macintosh
Syntax
Environ({envstring | number})
The Environ function syntax has these named arguments:
Part Description
envstring Optional. String expression containing the name of an environment variable.
number Optional. Numeric expression corresponding to the numeric order of the environment string in the environment-string table. The number argument can be any numeric expression, but is rounded to a whole number before it is evaluated.
Remarks
If envstring can't be found in the environment-string table, a zero-length string ("") is returned. Otherwise, Environ returns the text assigned to the specified envstring; that is, the text following the equal sign (=) in the environment-string table for that environment variable.
If you specify number, the string occupying that numeric position in the environment-string table is returned. In this case, Environ returns all of the text, including envstring. If there is no environment string in the specified position, Environ returns a zero-length string.
Sub List_Environment_Variables()
Dim EnvString As String
Indx = 1
Do
EnvString = Environ(Indx)
Cells(Indx, 1) = EnvString
Indx = Indx + 1
Loop Until EnvString = ""
End Sub
ALLUSERSPROFILE=C:\Documents and Settings\All Users
APPDATA=C:\Documents and Settings\nbdyvek\Application Data
CommonProgramFiles=C:\Program Files\Common Files
COMPUTERNAME=B0024811EE6F0
ComSpec=C:\WINNT\system32\cmd.exe
DEFLOGDIR=C:\Documents and Settings\All Users\Application Data\McAfee\DesktopProtection
FP_NO_HOST_CHECK=NO
HOMEDRIVE=C:
HOMEPATH=\Documents and Settings\nbdyvek
LOGONSERVER=\\ASIGGNDCS00
LSERVRC=C:\Program Files\Common Files\Mercury Interactive\License Manager\lservrc
LSHOST=172.23.3.194
NUMBER_OF_PROCESSORS=4
OS=Windows_NT
Path=C:\Program Files\Microsoft Office\Office12\;c:\Program Files\oracle\product\10.2.0\client_1\bin;C:\WINNT\system32;C:\WINNT;C:\WINNT\System32\Wbem;"C:\Program Files\PKWARE\pkzipc"
PATHEXT=.COM;.EXE;.BAT;.CMD;.VBS;.VBE;.JS;.JSE;.WSF;.WSH
PROCESSOR_ARCHITECTURE=x86
PROCESSOR_IDENTIFIER=x86 Family 6 Model 23 Stepping 10, GenuineIntel
PROCESSOR_LEVEL=6
PROCESSOR_REVISION=170a
ProgramFiles=C:\Program Files
SESSIONNAME=Console
SystemDrive=C:
SystemRoot=C:\WINNT
TEMP=C:\DOCUME~1\nbdyvek\LOCALS~1\Temp
TMP=C:\DOCUME~1\nbdyvek\LOCALS~1\Temp
USERDNSDOMAIN=ASIA.BANKOFAMERICA.COM
USERDOMAIN=ASIA
USERNAME=nbdyvek
USERPROFILE=C:\Documents and Settings\nbdyvek
VSEDEFLOGDIR=C:\Documents and Settings\All Users\Application Data\McAfee\DesktopProtection
WecVersionForRosebud.E88=3
windir=C:\WINNT
Connecting to MS Access DataBase using QTP
Dim rs,con
set con = createobject("adodb.connection")
set rs = createobject("adodb.recordset")
con.provider = "microsoft.jet.oledb.4.0"
con.open "C:\Program Files\Mercury Interactive\QuickTest Professional\samples\flight\app\flight32.mdb"
rs.open "select * from Orders where Flight_Number=4219 ",con
'to know the number of orders in class1
'rs.open "select count(*) from Orders group by class",con
'to insert into database
rs.open "insert into emp(empno,empname,empsal) values (4,'lakshmi',400),con
'display each field in the record set
Do while not rs.eof
For Each Field In rs.Fields
MsgBox Field.name
MsgBox Field.value
Next
rs.MoveNext
Loop
' Close the connection.
con.Close
set con = createobject("adodb.connection")
set rs = createobject("adodb.recordset")
con.provider = "microsoft.jet.oledb.4.0"
con.open "C:\Program Files\Mercury Interactive\QuickTest Professional\samples\flight\app\flight32.mdb"
rs.open "select * from Orders where Flight_Number=4219 ",con
'to know the number of orders in class1
'rs.open "select count(*) from Orders group by class",con
'to insert into database
rs.open "insert into emp(empno,empname,empsal) values (4,'lakshmi',400),con
'display each field in the record set
Do while not rs.eof
For Each Field In rs.Fields
MsgBox Field.name
MsgBox Field.value
Next
rs.MoveNext
Loop
' Close the connection.
con.Close
How to select radio buttons
For a Windows radio button which is not in a group the following is the syntax:
"Set" method : sets the radio button as the selected radio button.
Window("Flight Reservation").WinRadioButton("Business").Set
For a Windows radio button which is in a group the following is the syntax:
Select Selects the specified radio button from the radio group, replacing the previous selection.
e.g.Browser("Google").Page("Google").WebRadioGroup("meta").Select "cr=countryIN"
"Set" method : sets the radio button as the selected radio button.
Window("Flight Reservation").WinRadioButton("Business").Set
For a Windows radio button which is in a group the following is the syntax:
Select Selects the specified radio button from the radio group, replacing the previous selection.
e.g.Browser("Google").Page("Google").WebRadioGroup("meta").Select "cr=countryIN"
Wednesday, July 14, 2010
Difference between Updated Run Mode & Maintenance Run Mode?
Update Run mode updates all properties of objects and checkpoints in case of failures. So if a object gets recognized using SMart identification then correct properties are updated in the OR. In case expected and actual values of checkpoints are different then they are updated as per the actuals.It can be selected from Automation -> Update Run mode
Maintenance run mode is used when you want to update objects which are failing during execution. So if the object cannot be recognized at all with current settings instead of giving an error QTP will give a dialog to point the object to a new object if required.
Sunday, July 4, 2010
What is the Automation Framework ?
A framework is an integrated system or an execution environment that sets the rules of Automation of a specific product.
The framework provides the basis of test automation and simplifies the automation effort.
This system integrates the function libraries, test data sources, object details and various reusable modules. These components act as small building blocks which need to be assembled to represent a business process.
There are various types of frameworks. They are categorized on the basis of the automation component they leverage. These are:
Data-driven testing :
Testing of application with multiple sets of data.The disadvatage with this framework is its Application dependent scripts.
Keyword-driven testing :
Keyword-driven testing: In Keyword Driven Framework every keyword represents some code which will perform certain action on the application.We create the excel file in which we provide the steps in simple english words (Keywords) by the user.
Advantage:The advantages for automated tests are the reusability and therefore ease of maintenance of tests that have been created at a high level of abstraction.
Disadvantage: Even for small actions in test Data file we need to write multiple lines to achieve the functionality.
Action Driven framework:
It takes the advantages for the Data Driven and Keyword Driven framework and automates thte application.Every Action here means a specific script which will perform only certain action provided the data in test data file.In RealTime people often call this Hybrid testing.
Hybrid testing :
The hybrid Test Automation Framework is what most frameworks evolve into over time and multiple projects. The most successful automation frameworks generally accommodate both Keyword-driven testing as well as Data-driven testing. This allows data driven scripts to take advantage of the powerful libraries and utilities that usually accompany a keyword driven architecture
The framework provides the basis of test automation and simplifies the automation effort.
This system integrates the function libraries, test data sources, object details and various reusable modules. These components act as small building blocks which need to be assembled to represent a business process.
There are various types of frameworks. They are categorized on the basis of the automation component they leverage. These are:
Data-driven testing :
Testing of application with multiple sets of data.The disadvatage with this framework is its Application dependent scripts.
Keyword-driven testing :
Keyword-driven testing: In Keyword Driven Framework every keyword represents some code which will perform certain action on the application.We create the excel file in which we provide the steps in simple english words (Keywords) by the user.
Advantage:The advantages for automated tests are the reusability and therefore ease of maintenance of tests that have been created at a high level of abstraction.
Disadvantage: Even for small actions in test Data file we need to write multiple lines to achieve the functionality.
Action Driven framework:
It takes the advantages for the Data Driven and Keyword Driven framework and automates thte application.Every Action here means a specific script which will perform only certain action provided the data in test data file.In RealTime people often call this Hybrid testing.
Hybrid testing :
The hybrid Test Automation Framework is what most frameworks evolve into over time and multiple projects. The most successful automation frameworks generally accommodate both Keyword-driven testing as well as Data-driven testing. This allows data driven scripts to take advantage of the powerful libraries and utilities that usually accompany a keyword driven architecture
What is Test Automation ?
Although manual tests may find many defects in a software application, it is a laborious and time consuming process. In addition it may not be effective in finding certain classes of defects. Test automation is a process of writing a computer program to do testing that would otherwise need to be done manually. Once tests have been automated, they can be run quickly. This is often the most cost effective method for software products that have a long maintenance life, because even minor patches over the lifetime of the application can cause features to break which were working at an earlier point in time.
Do you prefer to work alone or as a team ?
I would like to work in an environment where there are both the sides.
Its great working with team and sharing the knowledge, and learning ideas with each other.
It's also great to sit at my own desk and work independently towards team goal.
Its great working with team and sharing the knowledge, and learning ideas with each other.
It's also great to sit at my own desk and work independently towards team goal.
Sunday, June 27, 2010
What is consumer finance?
The division of retail banking that deals with lending money to consumers. This includes a wide variety of loans, including credit cards, mortgage loans, and auto loans, and can also be used to refer to loans taken out at either the prime rate or the subprime rate.
Prime rate or prime lending rate is a term applied in many countries to a reference interest rate used by banks. The term originally indicated the rate of interest at which banks lent to favored customers, i.e., those with high credibility, though this is no longer always the case. Some variable interest rates may be expressed as a percentage above or below prime rate.
subprime lending means making loans that are in the riskiest category of consumer loans and are typically sold in a separate market from prime loans.
Prime rate or prime lending rate is a term applied in many countries to a reference interest rate used by banks. The term originally indicated the rate of interest at which banks lent to favored customers, i.e., those with high credibility, though this is no longer always the case. Some variable interest rates may be expressed as a percentage above or below prime rate.
subprime lending means making loans that are in the riskiest category of consumer loans and are typically sold in a separate market from prime loans.
Friday, June 25, 2010
Delete duplicate records from a table
delete from test a
where rowid <> ( select max(rowid)
from test b
where a.sno = b.sno
and a.sname = b.sname )
where rowid <> ( select max(rowid)
from test b
where a.sno = b.sno
and a.sname = b.sname )
Monday, June 21, 2010
How to work with APIs or DLLs in Excel VBA ?
An API is exactly as its acronym suggests, an interface. Just like the interface between your screen and yourself is the keyboard or mouse, so the underlying code just translates your actions to the screen....everything between these 2 is handled by Library files that call other functions to perform actions. You use API while programing in VBA, you just don't realise it...the VBA just acts like a translator in converting your code to the appropriate Op-codes so that the underlying library files can perform some action/function.
The plus side of using API's means that the libraries can be updated independently of the application, and many applications can share a single DLL. The down side is that if you get it wrong you may not receive an error and/or your system will become unstable or crash causing you to reboot.
Always good practice to constantly save when working with APIs. Also can be difficult to debug your program, in fact you can't debug API calls in VBA.
In VBA before you can call a function in a DLL, you must provide VBA with information about where that function is and how to call it. There are two ways to do this:
1) by setting a reference to the DLL's type library (.tlb)
2) by using a Declare statement in a module as above.
The Declare keyword alerts VBA that you want to include the definition for a DLL function in your project. A Declare statement in a standard module can be public or private, depending on whether you want the API function to be available to only a single module or to the entire project. For class modules, a Declare statement must be private so .........................
The Public Function >
Public Declare Function sndPlaySound Lib "winmm.dll" _
Alias "sndPlaySoundA" ( _
ByVal lpszSoundName As String, _
ByVal uFlags As Long) As Long
In most cases you will see API calls with No explicit path as you would use the core window library files which are located in your system dir.
If a procedure does not return a value, write the declare as a Sub:
eg.
Declare Sub publicname Lib "libname" [Alias "alias"] [([[ByVal] variable [As type] [,[ByVal] variable [As type]]...])]
As per Std Sub Procedure set-up, DLL procedures declared in standard modules are public by default and can be called from anywhere in your application. DLL procedures declared in any other type of module are private to that module, and you must identify them as such by preceding the declaration with the Private keyword. Procedure names are case-sensitive in 32-bit versions of Visual Basic. In previous, 16-bit versions, procedure names were not case-sensitive.
Lets look @ the above Function;
[Lib]
Specifying the Library
The Lib clause in the Declare statement tells Visual Basic where to find the .dll file that contains the procedure. When you're referencing one of the core Windows libraries;
User32 [user interface functions],
Kernel32 [operating system kernel functions],
GDI32 [graphics device interface functions], and
shell32.dll [Windows shell functions],
you don't need to include the file name extension:
eg.
Declare Function RegisterClass Lib "user32" Alias "RegisterClass" (Class As WNDCLASS) As Long
For other DLLs, the Lib clause is a file specification that can include a path:
Declare Function lzCopy Lib "c:\windows\lzexpand.dll"
_(ByVal S As
_ Integer, ByVal D As Integer) As Long
If you do not specify a path for libname, Visual Basic will search for the file in the following order:
1. Directory containing the .exe file
2. Current directory
3. Windows 32-bit system directory \Windows\System32
4. Windows directory \Windows
5. Path environment variable (See here to get these)
In most cases you will see API calls with No explicit path as you would use the core window library files which are located in your system dir. So in the above example we are Looking @ the winmm.dll
sndPlaySound = The name by which Visual Basic will refer to the function everywhere else in your code. You can make this any name you want (as long as you use the proper Alias clause), but it's safest to make this the Actual name of the function in the Windows API.
[Alias]
The Alias clause in the declare statement is needed to specify the correct character set. Windows API functions that contain strings actually exist in two formats: ANSI and Unicode.
It is the A (ANSI) @ end that signifies this in "sndPlaySoundA"
The Unicode version ends with the letter W (WCHAR type). So if the function has two entry points then you would expect to see the Alias used.
ANSI or Unicode
The 32-bit versions of Windows (Windows 95+ and Windows NT) support two separate character sets: ANSI, in which each character takes 1 byte of storage and there are only 256 different possible characters; and Unicode, in which each character takes up 2 bytes of storage and there are 65,536 different possible characters ie. 16-bit Unicode character. VBA, Access' programming language, supports Unicode only. In general, this works in your favour, allowing support for many languages, including those that use character sets different from the English ones. In the Windows header files, therefore, you'll get both ANSI and Unicode versions of each function that contains a string. Have a look for winmm.dll and right click to select Quickview, you should get something like this;
Export table
Ordinal | Entry Point | Name
0004 00012d0 CloseDriver
0005 0008db3 DefDriverProc
etc....listing all the entry points or LP Long pointers to the various functions with in the library.
Now for the Arguments and Types;
(ByVal lpszSoundName As String, ByVal uFlags As Long) As Long
lpszSoundName = a string that specifies the sound to play. This must be the Fullpathname. If this parameter is NULL, any currently playing waveform sound is stopped. ie To stop a currently playing sound then send the VBA Const VBNullString and NOT "" !!
ByVal = when a value is passed ByVal, the actual value is passed directly to the function, the exception to this is string variables, but that is another story, and when passed ByRef, the address of the value is passed, so you may see something like LPWORD which is actually a Long Pointer to a memory address.
As String = value passed is a string = the full path name to the sound file
ByVal uFlags As Long
The function is expecting some Flags to be declared here as the data type Long (Look up data Type for Long) = Long (long integer) variables are stored as signed 32-bit (4-byte) numbers ranging in value from -2,147,483,648 to 2,147,483,647. The type-declaration character for Long is the ampersand (&).
As for the Public Constants
Public Const SND_SYNC = &H0
Public Const SND_ASYNC = &H1
Public Const SND_NODEFAULT = &H2
Public Const SND_MEMORY = &H4
Public Const SND_LOOP = &H8
Public Const SND_NOSTOP = &H10
What does &H0, &H1, &H2, &H4, &H8, &H10 mean?? They are Hexadecimal values (Base 16)
The function will recognize various Constants or flags that tell it how/what to do eg.
Flags for playing the sound;
SND_ASYNC
The sound is played asynchronously and PlaySound returns immediately after beginning the sound. To terminate an asynchronously played waveform sound, call PlaySound with lpszSoundName set to VBNullString.
SND_LOOP
The sound plays repeatedly until PlaySound is called again with the lpszSoundName parameter set to VBNullString. You must also specify the SND_ASYNC flag to indicate an asynchronous sound event.
SND_NODEFAULT
No default sound event is used. If the sound cannot be found, PlaySound returns silently without playing the default sound.
SND_NOSTOP
The specified sound event will yield to another sound event that is already playing. If a sound cannot be played because the resource needed to generate that sound is busy playing another sound,the function immediately returns FALSE without playing the requested sound. If this flag is not specified, PlaySound attempts to stop the currently playing sound so that the device can be used to play the new sound.
SND_NOWAIT
If the driver is busy, then return immediately without playing the sound.
SND_SYNC
Synchronous playback of a sound event. PlaySound returns after the sound event completes.
The values; &H0, &H1, &H2, &H4, &H8, &H10 are the Long Hex values of these constants eg.
&[Long] H(Hex) 0 or &H0 = 0 Decimal
&[Long] H(Hex) 10 or &H10 = 16 Decimal
The plus side of using API's means that the libraries can be updated independently of the application, and many applications can share a single DLL. The down side is that if you get it wrong you may not receive an error and/or your system will become unstable or crash causing you to reboot.
Always good practice to constantly save when working with APIs. Also can be difficult to debug your program, in fact you can't debug API calls in VBA.
In VBA before you can call a function in a DLL, you must provide VBA with information about where that function is and how to call it. There are two ways to do this:
1) by setting a reference to the DLL's type library (.tlb)
2) by using a Declare statement in a module as above.
The Declare keyword alerts VBA that you want to include the definition for a DLL function in your project. A Declare statement in a standard module can be public or private, depending on whether you want the API function to be available to only a single module or to the entire project. For class modules, a Declare statement must be private so .........................
The Public Function >
Public Declare Function sndPlaySound Lib "winmm.dll" _
Alias "sndPlaySoundA" ( _
ByVal lpszSoundName As String, _
ByVal uFlags As Long) As Long
In most cases you will see API calls with No explicit path as you would use the core window library files which are located in your system dir.
If a procedure does not return a value, write the declare as a Sub:
eg.
Declare Sub publicname Lib "libname" [Alias "alias"] [([[ByVal] variable [As type] [,[ByVal] variable [As type]]...])]
As per Std Sub Procedure set-up, DLL procedures declared in standard modules are public by default and can be called from anywhere in your application. DLL procedures declared in any other type of module are private to that module, and you must identify them as such by preceding the declaration with the Private keyword. Procedure names are case-sensitive in 32-bit versions of Visual Basic. In previous, 16-bit versions, procedure names were not case-sensitive.
Lets look @ the above Function;
[Lib]
Specifying the Library
The Lib clause in the Declare statement tells Visual Basic where to find the .dll file that contains the procedure. When you're referencing one of the core Windows libraries;
User32 [user interface functions],
Kernel32 [operating system kernel functions],
GDI32 [graphics device interface functions], and
shell32.dll [Windows shell functions],
you don't need to include the file name extension:
eg.
Declare Function RegisterClass Lib "user32" Alias "RegisterClass" (Class As WNDCLASS) As Long
For other DLLs, the Lib clause is a file specification that can include a path:
Declare Function lzCopy Lib "c:\windows\lzexpand.dll"
_(ByVal S As
_ Integer, ByVal D As Integer) As Long
If you do not specify a path for libname, Visual Basic will search for the file in the following order:
1. Directory containing the .exe file
2. Current directory
3. Windows 32-bit system directory \Windows\System32
4. Windows directory \Windows
5. Path environment variable (See here to get these)
In most cases you will see API calls with No explicit path as you would use the core window library files which are located in your system dir. So in the above example we are Looking @ the winmm.dll
sndPlaySound = The name by which Visual Basic will refer to the function everywhere else in your code. You can make this any name you want (as long as you use the proper Alias clause), but it's safest to make this the Actual name of the function in the Windows API.
[Alias]
The Alias clause in the declare statement is needed to specify the correct character set. Windows API functions that contain strings actually exist in two formats: ANSI and Unicode.
It is the A (ANSI) @ end that signifies this in "sndPlaySoundA"
The Unicode version ends with the letter W (WCHAR type). So if the function has two entry points then you would expect to see the Alias used.
ANSI or Unicode
The 32-bit versions of Windows (Windows 95+ and Windows NT) support two separate character sets: ANSI, in which each character takes 1 byte of storage and there are only 256 different possible characters; and Unicode, in which each character takes up 2 bytes of storage and there are 65,536 different possible characters ie. 16-bit Unicode character. VBA, Access' programming language, supports Unicode only. In general, this works in your favour, allowing support for many languages, including those that use character sets different from the English ones. In the Windows header files, therefore, you'll get both ANSI and Unicode versions of each function that contains a string. Have a look for winmm.dll and right click to select Quickview, you should get something like this;
Export table
Ordinal | Entry Point | Name
0004 00012d0 CloseDriver
0005 0008db3 DefDriverProc
etc....listing all the entry points or LP Long pointers to the various functions with in the library.
Now for the Arguments and Types;
(ByVal lpszSoundName As String, ByVal uFlags As Long) As Long
lpszSoundName = a string that specifies the sound to play. This must be the Fullpathname. If this parameter is NULL, any currently playing waveform sound is stopped. ie To stop a currently playing sound then send the VBA Const VBNullString and NOT "" !!
ByVal = when a value is passed ByVal, the actual value is passed directly to the function, the exception to this is string variables, but that is another story, and when passed ByRef, the address of the value is passed, so you may see something like LPWORD which is actually a Long Pointer to a memory address.
As String = value passed is a string = the full path name to the sound file
ByVal uFlags As Long
The function is expecting some Flags to be declared here as the data type Long (Look up data Type for Long) = Long (long integer) variables are stored as signed 32-bit (4-byte) numbers ranging in value from -2,147,483,648 to 2,147,483,647. The type-declaration character for Long is the ampersand (&).
As for the Public Constants
Public Const SND_SYNC = &H0
Public Const SND_ASYNC = &H1
Public Const SND_NODEFAULT = &H2
Public Const SND_MEMORY = &H4
Public Const SND_LOOP = &H8
Public Const SND_NOSTOP = &H10
What does &H0, &H1, &H2, &H4, &H8, &H10 mean?? They are Hexadecimal values (Base 16)
The function will recognize various Constants or flags that tell it how/what to do eg.
Flags for playing the sound;
SND_ASYNC
The sound is played asynchronously and PlaySound returns immediately after beginning the sound. To terminate an asynchronously played waveform sound, call PlaySound with lpszSoundName set to VBNullString.
SND_LOOP
The sound plays repeatedly until PlaySound is called again with the lpszSoundName parameter set to VBNullString. You must also specify the SND_ASYNC flag to indicate an asynchronous sound event.
SND_NODEFAULT
No default sound event is used. If the sound cannot be found, PlaySound returns silently without playing the default sound.
SND_NOSTOP
The specified sound event will yield to another sound event that is already playing. If a sound cannot be played because the resource needed to generate that sound is busy playing another sound,the function immediately returns FALSE without playing the requested sound. If this flag is not specified, PlaySound attempts to stop the currently playing sound so that the device can be used to play the new sound.
SND_NOWAIT
If the driver is busy, then return immediately without playing the sound.
SND_SYNC
Synchronous playback of a sound event. PlaySound returns after the sound event completes.
The values; &H0, &H1, &H2, &H4, &H8, &H10 are the Long Hex values of these constants eg.
&[Long] H(Hex) 0 or &H0 = 0 Decimal
&[Long] H(Hex) 10 or &H10 = 16 Decimal
How to pause the execution of vba script?
Public Sub
‘Stops the execution of the code and continues after 10 seconds.
Application.Wait Now + TimeValue("00:00:10")
End sub
‘Stops the execution of the code and continues after 10 seconds.
Application.Wait Now + TimeValue("00:00:10")
End sub
Monday, June 14, 2010
How will you delete duplicate rows from a table?
DELETE FROM our_table
WHERE rowid not in
(SELECT MIN(rowid)
FROM our_table
GROUP BY column1, column2, column3... ;Here column1, column2, column3 constitute the identifying key for each record.
Be sure to replace our_table with the table name from which you want to remove the duplicate rows. The GROUP BY is used on the columns that make the primary key for the table. This script deletes each row in the group after the first row.
e.g.
DELETE FROM duptest WHERE rowid NOT IN (SELECT max(rowid) FROM duptest GROUP BY empid);
Explanation :- In the subquery I Select a unique rowid from all the rowid's It can be MAX, MIN any one and the group by clause should include all the UNIQUE columns I desire. Say I want a composite primary key then the group by should be group by id1,id2 ...
The the subquery returns one record for the dupliacte ID's and I delete all of them that are not in the subquery and that deletes all the dupliacte rows from the database.
WHERE rowid not in
(SELECT MIN(rowid)
FROM our_table
GROUP BY column1, column2, column3... ;Here column1, column2, column3 constitute the identifying key for each record.
Be sure to replace our_table with the table name from which you want to remove the duplicate rows. The GROUP BY is used on the columns that make the primary key for the table. This script deletes each row in the group after the first row.
e.g.
DELETE FROM duptest WHERE rowid NOT IN (SELECT max(rowid) FROM duptest GROUP BY empid);
Explanation :- In the subquery I Select a unique rowid from all the rowid's It can be MAX, MIN any one and the group by clause should include all the UNIQUE columns I desire. Say I want a composite primary key then the group by should be group by id1,id2 ...
The the subquery returns one record for the dupliacte ID's and I delete all of them that are not in the subquery and that deletes all the dupliacte rows from the database.
How to count number of rows in a text file ?
Const ForReading = 1, ForWriting = 2, ForAppending = 8
Dim objfile
Set objfile = CreateObject("Scripting.FileSystemObject")
Set objref = objfile.opentextfile("C:\\Results.txt", ForReading, True)
i = 0
Do While objref.AtEndOfStream <> True
TextLine = objref.ReadLine
i = i + 1
Loop
MsgBox i
Dim objfile
Set objfile = CreateObject("Scripting.FileSystemObject")
Set objref = objfile.opentextfile("C:\\Results.txt", ForReading, True)
i = 0
Do While objref.AtEndOfStream <> True
TextLine = objref.ReadLine
i = i + 1
Loop
MsgBox i
QTP Interview Questions
QTP Interview Questions can be found in this blog.Feel free to post or ask any questions on QTP.
Thursday, June 10, 2010
delete the highest earning employees in each department
SQL> select * from dept order by deptno;
DEPTNO DNAME LOC
---------- -------------- -------------
10 ACCOUNTING NEW YORK
20 RESEARCH DALLAS
30 SALES CHICAGO
40 OPERATIONS BOSTON
SQL> select empno,ename,job,sal,deptno from emp;
EMPNO ENAME JOB SAL DEPTNO
---------- ---------- --------- ---------- ----------
7369 SMITH CLERK 800 20
7499 ALLEN SALESMAN 1600 30
7521 WARD SALESMAN 1250 30
7566 JONES MANAGER 2975 20
7654 MARTIN SALESMAN 1250 30
7698 BLAKE MANAGER 2850 30
7782 CLARK MANAGER 2450 10
7788 SCOTT ANALYST 3000 20
7839 KING PRESIDENT 5000 10
7844 TURNER SALESMAN 1500 30
7876 ADAMS CLERK 1100 20
7900 JAMES CLERK 950 30
7902 FORD ANALYST 3000 20
7934 MILLER CLERK 1300 10
14 rows selected.
SQL> delete from emp a where
a.sal = (select max(sal) from emp b
where a.deptno = b.deptno);
4 rows deleted.
SQL> select ename, sal, deptno from emp
order by deptno, ename;
ENAME SAL DEPTNO
---------- ---------- ----------
CLARK 2916.67 10
MILLER 2916.67 10
ADAMS 2175 20
JONES 2975 20
SMITH 2175 20
ALLEN 1600 30
JAMES 1566.67 30
MARTIN 1566.67 30
TURNER 1566.67 30
WARD 1566.67 30
DEPTNO DNAME LOC
---------- -------------- -------------
10 ACCOUNTING NEW YORK
20 RESEARCH DALLAS
30 SALES CHICAGO
40 OPERATIONS BOSTON
SQL> select empno,ename,job,sal,deptno from emp;
EMPNO ENAME JOB SAL DEPTNO
---------- ---------- --------- ---------- ----------
7369 SMITH CLERK 800 20
7499 ALLEN SALESMAN 1600 30
7521 WARD SALESMAN 1250 30
7566 JONES MANAGER 2975 20
7654 MARTIN SALESMAN 1250 30
7698 BLAKE MANAGER 2850 30
7782 CLARK MANAGER 2450 10
7788 SCOTT ANALYST 3000 20
7839 KING PRESIDENT 5000 10
7844 TURNER SALESMAN 1500 30
7876 ADAMS CLERK 1100 20
7900 JAMES CLERK 950 30
7902 FORD ANALYST 3000 20
7934 MILLER CLERK 1300 10
14 rows selected.
SQL> delete from emp a where
a.sal = (select max(sal) from emp b
where a.deptno = b.deptno);
4 rows deleted.
SQL> select ename, sal, deptno from emp
order by deptno, ename;
ENAME SAL DEPTNO
---------- ---------- ----------
CLARK 2916.67 10
MILLER 2916.67 10
ADAMS 2175 20
JONES 2975 20
SMITH 2175 20
ALLEN 1600 30
JAMES 1566.67 30
MARTIN 1566.67 30
TURNER 1566.67 30
WARD 1566.67 30
Tuesday, June 8, 2010
Correlated Subquery
A query is called correlated subquery when both the inner query and the outer query are interdependent. For every row processed by the inner query, the outer query is processed as well. The inner query depends on the outer query before it can be processed.
A correlated Oracle subquery is evaluated once FOR EACH ROW as opposed to a normal subquery which is evaluated only once for each table.
You can reference the outer query inside the correlated subquery using an alias which makes it so handy to use.
SQL> select ename ,sal ,deptno from emp a where
a.sal < (select avg(sal) from emp b
where a.deptno = b.deptno)
order by deptno;
ENAME SAL DEPTNO
---------- ---------- ----------
CLARK 2450 10
MILLER 1300 10
SMITH 800 20
ADAMS 1100 20
WARD 1250 30
MARTIN 1250 30
TURNER 1500 30
JAMES 950 30
8 rows selected.
NOTE:
1) You can nest as many queries you want but it is recommended not to nest more than 16 subqueries in oracle.
2) If a subquery is not dependent on the outer query it is called a non-correlated subquery.
A correlated Oracle subquery is evaluated once FOR EACH ROW as opposed to a normal subquery which is evaluated only once for each table.
You can reference the outer query inside the correlated subquery using an alias which makes it so handy to use.
SQL> select ename ,sal ,deptno from emp a where
a.sal < (select avg(sal) from emp b
where a.deptno = b.deptno)
order by deptno;
ENAME SAL DEPTNO
---------- ---------- ----------
CLARK 2450 10
MILLER 1300 10
SMITH 800 20
ADAMS 1100 20
WARD 1250 30
MARTIN 1250 30
TURNER 1500 30
JAMES 950 30
8 rows selected.
NOTE:
1) You can nest as many queries you want but it is recommended not to nest more than 16 subqueries in oracle.
2) If a subquery is not dependent on the outer query it is called a non-correlated subquery.
write sql query to get the employees names under manager
It has emp table and mgr table.
To query the EMP table for the direct reports to the employee KING, you could write the following:
select ename from emp e
where mgr in (select empno from emp where ename = 'KING');
To query the EMP table for the direct reports to the employee KING, you could write the following:
select ename from emp e
where mgr in (select empno from emp where ename = 'KING');
Monday, June 7, 2010
Software Development Life Cycle Model
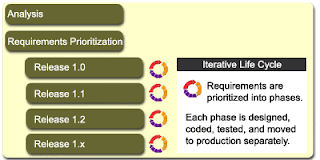
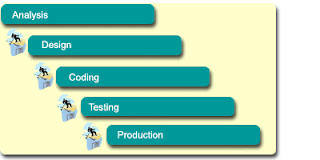
Waterfall method:
For years, the primary methodology for delivering software projects was the "Waterfall Method". With the Waterfall method, all software requirements are gathered up front, designs are done for each requirement, and each feature is coded and tested before migrating to production. For projects that exceed one year of development and implementation, there are risks of the project being cancelled (according to the Standish Group, about 31% are cancelled before completion).
Weaknesses of the Waterfall Method:
Since all requirements are gathered (and designs done) upfront, the software life cycle is normally a year or longer.
Business rules change along with market conditions. Due to the long software life cycle, the software may no longer meet the needs of the company after being implemented.
Testing is performed after all features are analyzed, designed and coded. If a major design flaw is found during the testing phase, it may need to be addressed in many functional areas, causing the time to fix the defect much longer due to the large number of requirements.
Iterative Development Model
An alternative approach is the Iterative Development Life Cycle (sometimes referred to as the Spiral Life Cycle).
With the Iterative Life Cycle, analysis is done just the same as with the Waterfall method. However, once analysis is done, each requirement is prioritized as follows:
High - These are mission critical requirements that absolutely have to be done in the first release.
Medium - These are requirements that are important but can be worked around until implemented.
Low - These are requirements that are nice-to-have but not critical to the operation of the software.
Once priorities have been established, the releases are planned. The first release (Release 1.0) will contain just the High priority items and should take about 1 to 3 months to deliver.
Below are the advantages of the Iterative Life Cycle:
The Design phase goes much faster, as designs are only done on the items in the current release (Release 1.0 for example).
Coding and Testing go much faster because there are less items to code and test. If major design flaws are found, re-work is much faster since the functional areas have been greatly reduced.
The client gets into production in less than 3 months, allowing them to begin earning revenue or reducing expenses quicker with their product.
If market conditions change for the client, changes can be incorporated in the next iterative release, allowing the software to be much more nimble.
As the software is implemented, the client can make recommendations for the next iteration due to experiences learned in the past iteration.
Our experience has found that you should space iterations at least 2 – 3 months a part. If iterations are closer than that, you spend too much time on convergence and the project timeframe expands. During the coding phase, code reviews must be done weekly to ensure that the developers are delivering to specification and all source code is put under source control. Also, full installation routines are to be used for each iterative release as it would be done in production.
Thursday, April 15, 2010
How to export the Search results in JIRA tool ?
1.Open the JIRA tool.
2.Select the required search criteria.
3.click on "View" button.
4.Under the "Current View" section click on Current Fields or All fields which will promot a save dialog .click save and specify the location.
2.Select the required search criteria.
3.click on "View" button.
4.Under the "Current View" section click on Current Fields or All fields which will promot a save dialog .click save and specify the location.
Subscribe to:
Posts (Atom)